Globe is an interface to handle all WebGL objects through a
Context. Globe is able :
- to draw layers,
- to render layers,
- to dispose layers and WebGL objects,
- to transform (longitude,latitude) from/to pixel coordinates
The pixel coordinates are expressed in the canvas frame, i.e. (0,0) corresponds to the lower-left corner of the pixel.
The geo-position coordinates(longitude,latitude) are expressed in decimal degree in their defined coordinate reference
system.
In Mizar, it exists two kinds of globes :
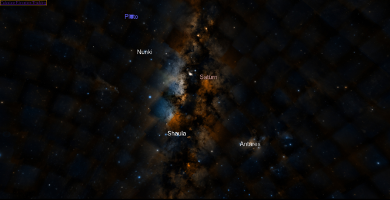 |
Sky |
Provides two grids to handle data according to their coordinate reference system : Equatorial, Galactic.
Then the globe renders the two grids in the same time. |
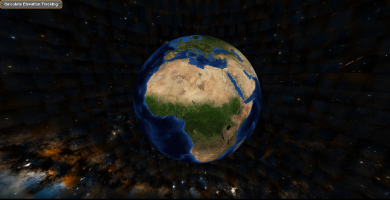 |
Planet |
Provides only one grid to handle data in the reference frame of the planet |
In addition to the classes, a
factory is available to help for creating
globe. Once the globe is created, the client can handle it by the use of its
interface.
- See:
-
-
isSky()
-
Returns:
True when the globe is a sky otherwise false
-
Type
-
boolean
-
getType()
-
Returns the type of globe.
Returns:
type of globe
-
Type
-
GLOBE
-
setBaseImagery(layer)
-
Registers the layer as a background raster and attach it to the globe.
When a raster layer is already set, this layer is replaced by the new one.
Parameters:
-
getBaseImagery()
-
Returns the background raster as a layer.
Returns:
the raster layer
-
Type
-
AbstractRasterLayer
-
setBaseElevation()
-
Registers the elevation layer and attach it to the globe.
When an elevation layer is already set, this layer is replaced by the new one
Returns:
the layer used
-
Type
-
WMSElevationLayer
|
WCSElevationLayer
-
getBaseElevation()
-
Returns the elevation layer.
Returns:
the layer used
-
Type
-
WMSElevationLayer
|
WCSElevationLayer
-
addLayer(layer)
-
Registers a new layer to globe to be visualized on the globe.
When the layer is added, an internal ID is generated to the layer based on an autoincrement value.
Once the layer is added, the globe is rendered. According to the visible attribute of the layer,
the layer is automatically shown on the globe.
The layer can be mainly a raster or a set of vectors.
In the vector case, the data is located in the url attribute of the layer object. In addition to the URL,
a callback attribute can be applied to the data.
Parameters:
Name |
Type |
Description |
layer |
Layer
|
the layer to add |
Fires:
-
removeLayer(layer)
-
Removes a layer.
The layer is set to unvisible. Then it is detached to the globe.
The globe is rendered to remove the layer from the globe.
Parameters:
Name |
Type |
Description |
layer |
Layer
|
the layer to remove |
Fires:
-
addAnimation(anim)
-
Adds an animation to be played later on.
Parameters:
Name |
Type |
Description |
anim |
Animation
|
the animation to add |
-
removeAnimation(anim)
-
Removes an animation.
Parameters:
Name |
Type |
Description |
anim |
Animation
|
the animation to remove |
-
getElevation(lon, lat)
-
Returns the elevation in meters at a geo position at the defined coordinate reference system.
Parameters:
Name |
Type |
Description |
lon |
float
|
the longitude in degree |
lat |
float
|
the latitude in degree |
Returns:
the elevation in meter at the position [lon,lat]
-
Type
-
float
-
getViewportGeoBound(transformCallback)
-
Returns the viewport geo bound.
Parameters:
Name |
Type |
Description |
transformCallback |
|
Callback transforming the frustum/globe intersection coordinates if needed |
Returns:
the geo bound of the viewport
-
Type
-
GeoBound
-
getLonLatFromPixel(x, y)
-
Returns the geo position [longitude, latitude] in degree from a pixel.
The pixel is expressed in the canvas frame, i.e. (0,0) corresponds to the lower-left corner of the pixel.
The geo position is expressed in the defined coordinate reference system.
Parameters:
Name |
Type |
Description |
x |
int
|
the pixel x coordinate |
y |
int
|
the pixel y coordinate |
Returns:
an array of two numbers [lon,lat] or null if the pixel is not on the globe
-
Type
-
Array.<float>
|
null
-
getPixelFromLonLat(lon, lat)
-
Returns the pixel coordinates from geo position [longitude, latitude] in degree in the defined coordinate reference
system.
The pixel is expressed in the canvas frame, i.e. (0,0) corresponds to the lower-left corner of the pixel
Parameters:
Name |
Type |
Description |
lon |
float
|
the longitude in decimal degree |
lat |
float
|
the latitude in decimal degree |
Returns:
an array of two numbers [x,y] or null if the pixel is not on the globe
-
Type
-
Array.<int>
|
null
-
setCoordinateSystem(coordinateSystem)
-
Sets the coordinate reference system
Parameters:
Name |
Type |
Description |
coordinateSystem |
Crs
|
the coordinate reference system |
-
getCoordinateSystem()
-
Returns the coordinate reference system.
Returns:
the coordinate reference system
-
Type
-
Crs
-
getRenderStats()
-
Displays some rendering statistics.
Returns:
the statistics
-
Type
-
string
-
getRenderContext()
-
Returns the rendering context.
Returns:
the rendering context
-
Type
-
RenderContext
-
setRenderContext(context)
-
Sets the rendering context.
Parameters:
Name |
Type |
Description |
context |
RenderContext
|
the rendering context |
-
getTileManager()
-
Returns the tile manager.
Returns:
Tile manager
-
Type
-
TileManager
-
dispose()
-
Cleans up every reference to gl objects and unloads all tiles
-
destroy()
-
Destroys the globe.
The globe is destroyed by :
- cleaning up every reference to gl objects and unloads all tiles
- Removing the renderer from all the tiles
-
refresh()
-
Refreshes rendering, must be called when canvas size is modified.
-
isEnabled()
-
Checks if the globe rendering is enabled.
Returns:
True when the globe rendering is enabled whereas False
-
Type
-
boolean
-
enable()
-
Enables the globe.
This is used to enable the sky when we use the sphere to map the data. In this way,
we see the globe and the sky in the same time.
-
disable()
-
Disables the globe.
This method is only overloaded by the sky to make disable the sky when the planet
is projected on a map.